Getting Started with EnquoDB and ActiveEnquo
This tutorial will walk you through creating a Ruby on Rails application that uses ActiveEnquo, an extension for ActiveRecord that provides advanced queryable encryption. We'll be building a simple application to store the names and dates of birth of people, and all that data will be encrypted and yet still queryable.
We assume that you've got Rails 6+ installed, on a Linux or macOS machine or VM.
Step 1: Create a Rails app, install gems
Naturally, if you're going to build a Rails app, the first thing to do is to create it.
Rails makes this easy, with the rails new command. So, run this in your command line (terminal app):
rails new active-enquo-demo -d postgresql
cd active-enquo-demo
ActiveEnquo and its dependencies are provided as pre-built RubyGems, so adding them to your new Rails application is as simple as adding the gem to your Gemfile and re-running bundler:
echo "gem 'active_enquo'" >> Gemfile
bundle install
If everything went well, then your new Rails app is now EnquoDB-enabled, and you can start to setup your database.
Step 2: Get an EnquoDB development database
ActiveEnquo uses a database server extension to perform queries. The easiest way to get started with ActiveEnquo is to create a free EnquoDB PostgreSQL development database. The sign up process will provision a database for you, and give you the details you need to add to your config/database.yml.
Alternately, you can install the pg_enquo extension in your own PostgreSQL database. Remember to configure config/database.yml appropriately.
Step 3: Setup an ActiveEnquo root key
ActiveEnquo derives all the encryption keys it needs from a single "root" key. This key should be kept well protected, as its disclosure would allow decryption of all data.
To generate an ActiveEnquo root key, run this command:
rails secret | cut -c 1-64
The output of that command is the hex-encoded key, which we now need to store in the Rails credentials vault, to keep it secure. To do this, open the credentials editor:
rails credentials:edit
Then add the following lines:
active_enquo:
root_key: "<paste hex-encoded key here>"
Step 4: Create a (scaffolded) table with encrypted columns
This is where the rubber meets the road. We'll use Rails' "scaffolding" feature to quickly create a database table and application functionality to manage the people in the table.
All EnquoDB column types start with enquo_, and the full list of available data types is available in the pg_enquo documentation. EnquoDB encrypted columns work mostly the same as built-in unencrypted column types. You can specify them in a generator like any other:
rails generate scaffold person \
first_name:enquo_text
last_name:enquo_text
date_of_birth:enquo_date
This will create all the necessary code to create the people table, and insert, read, and modify Person records.
You can now apply the generated migration to create the table:
rails db:migrate
Step 5: Run the app!
You now have enough to start using your new application. To start the application server, run:
rails s
If you visit http://localhost:3000/people in a browser, you should see a page something like this:
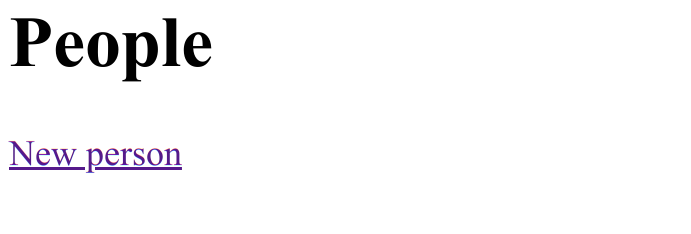
Clicking the "New person" link will provide you with a form that you can enter the details of a real or fictional person. Once you've filled in that form and clicked "Create Person", you'll see that the details are displayed decrypted in your browser. But are they securely stored in an encrypted form in the database?
Step 5½ (optional): Verify everything is encrypted
After you've got one or two records in your EnquoDB database, you can take a look at the contents of the people table to see that all of the data you've entered is, in fact, encrypted. To do this, get into the psql console by running:
rails dbconsole
Then ask for all records in the people table with this SQL:
SELECT * FROM people;
You will see that, rather than actual names and dates of birth, there is just large blobs of JSON. These JSON blobs are the ciphertexts of the data you have entered. Only a program with access to the configured root key can decrypt (and search) them.
Step 6: Query that data!
So far, everything we've done could have just as easily been accomplished with ActiveRecord Encryption. However, now that we're moving into the world of querying, we're going to do things that only EnquoDB can do.
Open up the file app/controllers/people.rb in your favourite editor, and modify the index method so that it looks like this:
def index
people = Person
%i{first_name last_name date_of_birth}.each do |field|
if params[field]
people = people.where(field => Person.enquo(field, params[field]))
end
end
@people = people.all
end
You can now filter the people which are returned by the /people endpoint by adding query parameters to the URL. For example, if you have a person whose first name is Jamie, you can list just those people by adding ?first_name=Jamie to the URL, like this:
http://localhost:3000/people?first_name=Jamie
Typically, adding query parameters is done using a HTML form. Adding such a form to your application is left as an exercise for the reader.
Step 7 (optional): Bulk data
If you'd like to try out EnquoDB on a larger data set, we've got a pre-compiled dataset of famous people and their birth dates you can load into your database. Assuming you've used the column names given above, this command line should be enough to load the data set into your database:
curl https://enquodb.com/examples/people.json | rails runner 'Person.create!(JSON.parse($stdin.read))'
This will load around 3,500 records into your people table. You'll now be able to find out, say, Neil Armstrong's birthday, by loading:
http://localhost:3000/people?first_name=Neil&last_name=Armstrong
Next Steps
You've now learnt the basics of using ActiveEnquo by building a simple dates-of-birth application. From here, you might want to try adding more fields to the table, or reference another table and add, say, "Notable Achievements".
Here's some more documentation that might help you out:
If you've got any questions or comments about this tutorial, we'd love to hear from you! Just e-mail contact@enquodb.com.